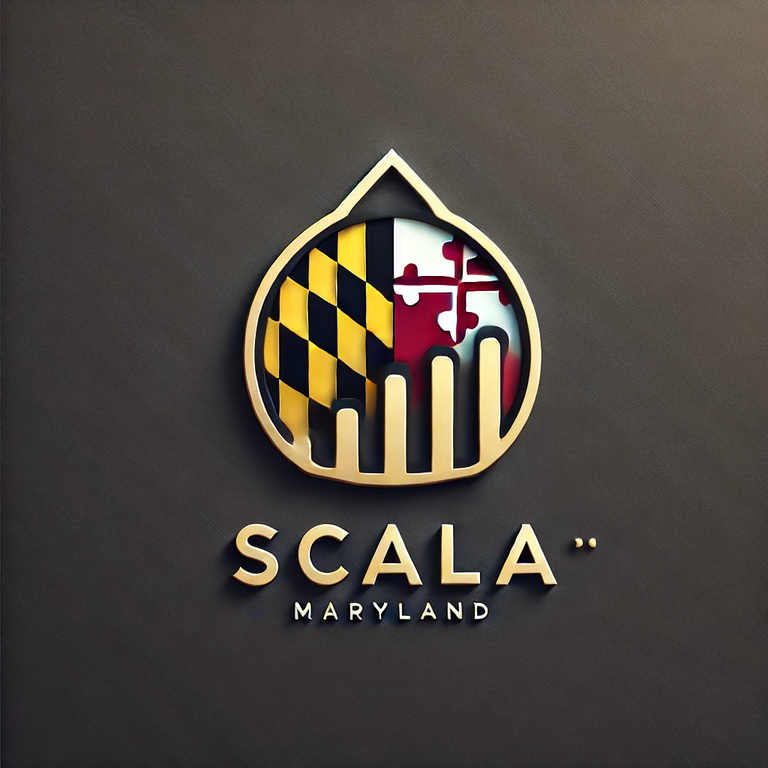
After coding my own things. I now I have profoundly greater respect for everyone keeping the software together for the rest of the populace whom have no idea.
The pure amount of code that it takes is absolutely insane to keep the world running.
I had to put this together to scan the wallet that will receive and withdrawal for the atomic swaps. I imagine the next phase is to find a way to track transactions and figure out the way to trigger transactions on Hive.... Wish me luck and give me some pointers.
import requests
import time
import sys
import datetime
# Scala Node
SCALA_NODE_URL = "http://remote.one.scala.network:11812/json_rpc"
# How many recent blocks to check?
BLOCK_SCAN_DEPTH = 50 # Scan last 50 blocks
# Your Scala Wallet Address
WALLET_ADDRESS = "Ssy2Eeyb1a1QGE5VW9kyQeEH2skMQxYfjjJ9Xua1w2VTGEgwKuzi4xZQu41SGDG5ab31Biqa8yhfHgTMTzHwACuT4DVjWfruWG"
def get_latest_block_height():
"""Fetch the latest block height."""
print("🔍 Requesting latest block height...", flush=True)
payload = {
"jsonrpc": "2.0",
"id": "0",
"method": "get_last_block_header"
}
response = requests.post(SCALA_NODE_URL, json=payload)
print(f"📡 Raw API response: {response.text}", flush=True)
if response.status_code == 200:
data = response.json()
if "result" in data and "block_header" in data["result"] and "height" in data["result"]["block_header"]:
block_height = data["result"]["block_header"]["height"]
print(f"🟢 Latest block height: {block_height}", flush=True)
return block_height
print("⚠️ Unexpected API response format:", data, flush=True)
return None
else:
print(f"❌ Error fetching latest block height (HTTP {response.status_code}): {response.text}", flush=True)
return None
def get_block_transactions(block_hash):
"""Retrieve full transaction details from a block."""
payload = {
"jsonrpc": "2.0",
"id": "0",
"method": "get_block",
"params": {"hash": block_hash}
}
response = requests.post(SCALA_NODE_URL, json=payload)
if response.status_code == 200:
data = response.json()
if "result" in data and "tx_hashes" in data["result"]:
transactions = []
for tx_hash in data["result"]["tx_hashes"]:
tx_data = get_transaction_details(tx_hash)
if tx_data:
transactions.append(tx_data)
return transactions
return []
def get_transaction_details(tx_hash):
"""Retrieve details of a specific transaction and check if it's related to our wallet."""
payload = {
"jsonrpc": "2.0",
"id": "0",
"method": "get_transaction",
"params": {"tx_hash": tx_hash}
}
response = requests.post(SCALA_NODE_URL, json=payload)
if response.status_code == 200:
data = response.json()
if "result" in data:
tx_data = data["result"].get("as_json", {})
# ✅ Extract outputs (recipients)
if "vout" in tx_data:
for output in tx_data["vout"]:
if "target" in output and "key" in output["target"]:
if output["target"]["key"] == WALLET_ADDRESS:
print(f"🚀 Transaction Found for Your Wallet! TxID: {tx_hash}")
return {
"tx_hash": tx_hash,
"amount": output["amount"],
"block_height": data["result"].get("block_height")
}
return None
def scan_recent_blocks():
"""Scan recent blocks for transactions."""
print(f"{datetime.datetime.now()} 🚀 Script started...", flush=True)
latest_height = get_latest_block_height()
if not latest_height:
print(f"{datetime.datetime.now()} ❌ Could not get latest block height. Exiting.", flush=True)
sys.exit(1)
print(f"{datetime.datetime.now()} ✅ Scanning the last {BLOCK_SCAN_DEPTH} blocks from height {latest_height}...", flush=True)
for i in range(BLOCK_SCAN_DEPTH):
block_height = latest_height - i
print(f"{datetime.datetime.now()} 🔍 Fetching block {block_height}...", flush=True)
block_payload = {
"jsonrpc": "2.0",
"id": "0",
"method": "get_block_header_by_height",
"params": {"height": block_height}
}
response = requests.post(SCALA_NODE_URL, json=block_payload)
print(f"{datetime.datetime.now()} 📡 Raw API response for block {block_height}: {response.text}", flush=True)
if response.status_code == 200:
data = response.json()
if "result" in data and "block_header" in data["result"] and "hash" in data["result"]["block_header"]:
block_hash = data["result"]["block_header"]["hash"]
print(f"{datetime.datetime.now()} 🟢 Block {block_height} has hash: {block_hash}", flush=True)
tx_hashes = get_block_transactions(block_hash)
if tx_hashes:
print(f"{datetime.datetime.now()} 🚀 Found {len(tx_hashes)} transactions in block {block_height}:", flush=True)
for tx in tx_hashes:
print(f"{datetime.datetime.now()} 🔹 Transaction Hash: {tx}", flush=True)
else:
print(f"{datetime.datetime.now()} ❌ No transactions found in block {block_height}", flush=True)
else:
print(f"{datetime.datetime.now()} ⚠️ Unexpected API response for block {block_height}: {data}", flush=True)
else:
print(f"{datetime.datetime.now()} ❌ HTTP Error {response.status_code} for block {block_height}: {response.text}", flush=True)
time.sleep(2)
if __name__ == "__main__":
scan_recent_blocks()