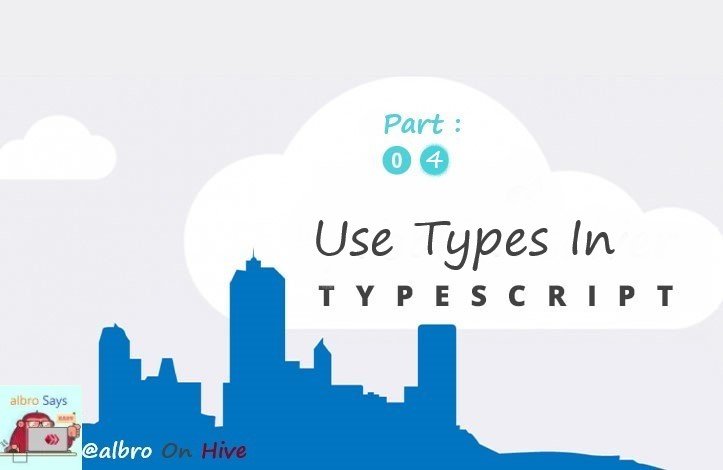
We worked on the following simple code:
function add(n1: number, n2: number) {
// if (typeof n1 !== 'number' || typeof n2 !== 'number') {
// throw new Error('Incorrect input!');
// }
return n1 + n2;
}
const number1 = 5;
const number2 = 2.8;
const result = add(number1, number2);
console.log(result);
Now suppose we add another constant to this code:
const number1 = 5; // 5.0
const number2 = 2.8;
const printResult = true;
The printResult
value is true
. I also want to print this Boolean
value inside my function, so I change the function to look like this:
function add(n1: number, n2: number, showResult: boolean) {
if (showResult) {
console.log(n1 + n2);
} else {
return n1 + n2;
}
}
In this code, I have first introduced a third parameter called showResult
, and then based on an if
condition, I have said that if showResult
is true
, log
the value of n1 + n2
, otherwise return
this value. Now I don't need to fix result and console.log
separately, because the add
function itself does this, so I change the end part of the code as follows:
add(number1, number2, printResult);
That is, I only call the function. Run tsc app.ts
again to update the javascript file. Now if you go to the browser you will see the value of 7.8
in the browser. Now let's make this code a little more complicated. I will add one more constant to our constants:
const number1 = 5; // 5.0
const number2 = 2.8;
const printResult = true;
const resultPhrase = 'Result is: ';
I want to put this string before displaying the number value, so I update the function again to accept a fourth argument:
function add(n1: number, n2: number, showResult: boolean, phrase: string) {
if (showResult) {
console.log(phrase + n1 + n2);
} else {
return n1 + n2;
}
}
What do you think the output of this function will be? Let's call the function to see the result:
add(number1, number2, printResult, resultPhrase);
Now if you go to the browser, you will see the result as follows:
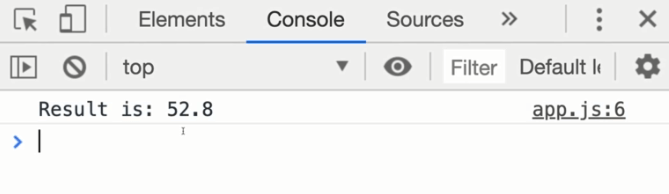
As you can see, our constant has been added successfully, but we have encountered the problem again and our numbers are added together as a string and show the result of 52.8
. why Because I have added a string with different numbers:
console.log(phrase + n1 + n2);
To solve this problem, we need to sum our numbers separately. For example:
function add(n1: number, n2: number, showResult: boolean, phrase: string) {
const result = n1 + n2;
if (showResult) {
console.log(phrase + result);
} else {
return result;
}
}
By adding the numbers separately, we prevent them from becoming strings. By running the tsc app.ts
command again, we see the following correct result in the browser:
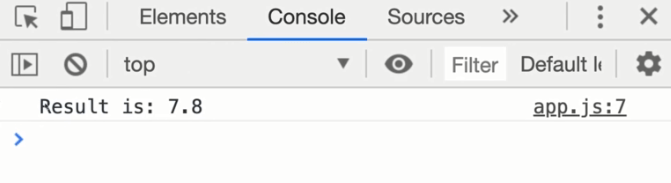
Now I have a question for you. Why didn't I specify the types when defining the constants? Shouldn't the type of variables be determined like parameters? In TypeScript, we can specify types when defining variables, not just parameters, but there is something called type inference in this language. Type inference means that the typescript itself guesses the type of your variable and assigns it to the variable. For example, if you move your mouse over the name of one of our constants, its type will be displayed:
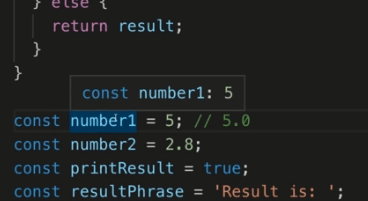
Since we used const
, our Type is not just a number
, but the exact number 5
! Because we are not allowed to change the value of const
s in JavaScript. If we use let
instead of const
and then move the mouse over it, it will show us the number
type:
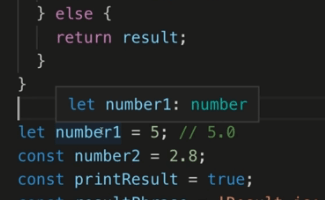
Of course, note that we can also manually define types for variables:
let number1: number = 5
Technically there is nothing wrong with the above code but you are advised not to do this as much as possible. The reason is that the typescript itself determines the type of the variable for you, so there is no need to overwrite it. The only case where this is recommended is when defining a variable without initialization:
let number1: number;
In this case, we tell the typescript what type of data this variable accepts. With this account the following code works without problems:
let number1: number;
number1 = 5;
But if we give it a string, we get an error:
let number1: number;
number1 = '5';
Certainly no one writes such code in their real program, I have used it for educational purposes only. This is because it is recommended to specify the type in non-valued variables. In a simple code like the one above, the mistake is obvious, but in real and large programs, a mistake may happen and a function in a part of the code passes a variable with the wrong type to another function, and our whole program crashes.
It also warns us if we want to change the type of the variable later. For example, if we change the constant resultPhrase
as follows:
let resultPhrase = 'Result is: ';
resultPhrase = 0;
We encounter an error because when defining the resultPhrase
, the type inference feature in the TypeScript language has set the type of the variable to "string
", but in the next line, we have changed this type to "number
". Since the typescript is a static type, it is not allowed to change the types and we encounter an error (a red line is drawn under the resultPhrase
). This is the task of typescript!
Until the next post, be happy!
[@albro on @hive]