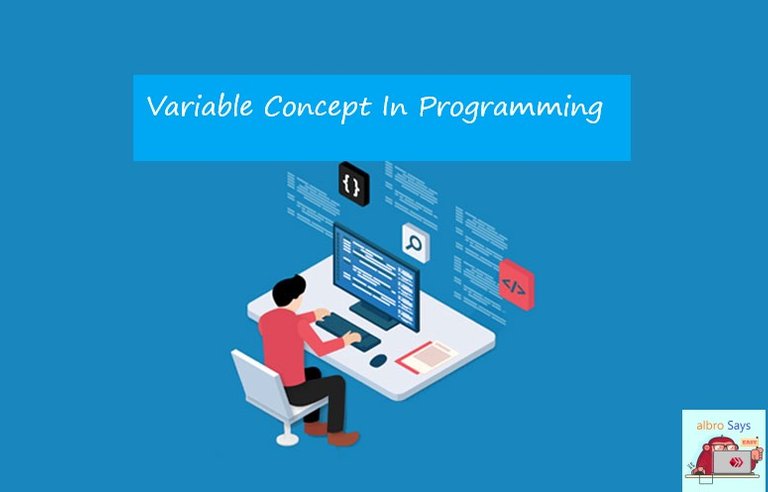
Variables in programming allow us to temporarily store various data. Each variable has a unique name in the program. Store the data we need in the variable so that we can access it later. In this post, regardless of the programming language, I will introduce the variable.
In very simple terms, a variable is a container that holds a value for us throughout the program.
Because we may have several variables during our programming, we name these containers (label). These labels are the same as the variable name. Whenever we need the stored value, we can use it by calling the variable name.
In this post, you will learn how to define variables in programming languages and how to store and call variables in the computer.
Variable In Programming
Suppose you want to write a program that takes two numbers from the user and performs two mathematical operations on them. After getting the first number, you cannot do the calculations and you have to wait for the second number. So we need to save the first number somewhere to use it later.
This is a very simple example of the use of variables in programming. Almost all programs that are more than a few dozen lines of code must have one or more variables.
At the beginning of the post, I said that we can consider the variable as a container. In order to have a container for storage, we must first create it, put something inside it, and finally use it when needed.
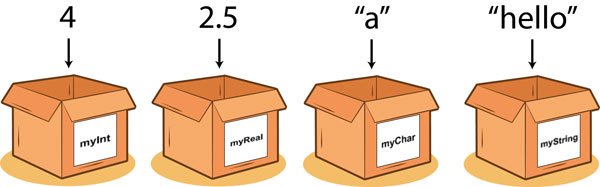
Look at programming variables as boxes
Steps To Work With Variables
So, defining and using variables in programming has three general steps:
- Defining Variable
- Variable Initialization
- Call the programming variable
When we want to define a variable, we must consider a name for it. This name must be unique throughout a program or within the scope of the program.
Scope means the range in which the variable is valid. This scope can be throughout the code (global variable) or, for example, within a programming function (local variable).
In some programming languages, it's necessary to specify the variable type. For example, we must specify whether this variable is used to store a number or a text string. I will talk more about this issue in the last section.
After defining the variable, we can put the desired value inside it. This can be done at the moment of variable definition or after.
The variable initialization operation is called variable assignment. In most languages, assignment is performed by the equal operator (=
).
Whenever we want to access the value stored in the variable, we just need to write the name of the variable in the program. In the pseudo code below, I have defined two variables x
and y
. y
is initialized at the same time as the definition, but x
is initialized in the third line. Finally, these two are added together and placed in the res
variable.
x
y = 11
x = 27
res = x + y
Variable naming in programming languages are similar. Most of them support English letters, numbers and the _
sign. To get familiar with the rules of your favorite language, refer to syntax rules and its introduction. I also suggest reading the variable naming standards (on this Wikipedia page).
Defining Programming Variables In Practice
The variable definition is different according to the syntax in each programming language. But the general method of variable definition in most high-level languages is as follows:
[< type >] < name > = < value >[;]
- If we don't want to initialize the variable, the
= < value >
part is removed from the code above. - In some programming languages, we don't need to specify the variable type. The type of the variable will be detected by the compiler or language interpreter. In these languages, the
< type >
section is not written. - In some languages like Python programming language, we don't need to put
;
at the end of each command line. - But in some languages such as PHP, Java or C programming language, we must put a
;
at the end of each command. In this case, this symbol is added to the end of the variable definition command.
How to define a variable in computer memory
As we all know, information is stored in the computer in two ways; random memory and long-term memory. The variables we use during programming are temporarily stored in the computer's main memory.
The amount of space that each type of variable occupies is different. The compiler or interpreter of the programming language determines how many bytes of space this variable needs in memory. Then it requests this space from the operating system. (One of the functions of the operating system is memory management.)
The operating system allocates one or more houses of memory to the program for the variable we defined.
In fact, the value stored in the variable name in the running program is the address of the first house from where the memory is allocated. This value can be different every time the program is run.
Types of variables in programming
The data types are briefly:
- Numerical data (correct and decimal)
- Text data (character and string)
- logical data
- Collection data type
- Structured data
Collection data in programming, generally known as collection, is a collection of several data in one variable.
For example, suppose you have a set of numbers that represent the grades of a student in a course.
The scores
variable is a variable, but 5 different numbers are placed inside it.
scores = [17.5, 15, 16.25, 19, 18.75]
The collection variable in different programming languages can have only one data type (only number, only text, etc.) or consist of several types together.
Collection data structures are known by different names and with different implementations. Some of the most used are array and list.
Structured data is data that is usually used by programmers to store information about a specific entity. For example, consider a variable that stores the information of a person. This structure has the characteristics of "name", "date of birth", "national code" and...
Structured variables are mostly used in the discussion of object-oriented programming.