This Dev Diary follows my post @cocaaladioxine/the-challenge-of-starting-a . I'll explain the main steps, choices and struggles while developing my application.
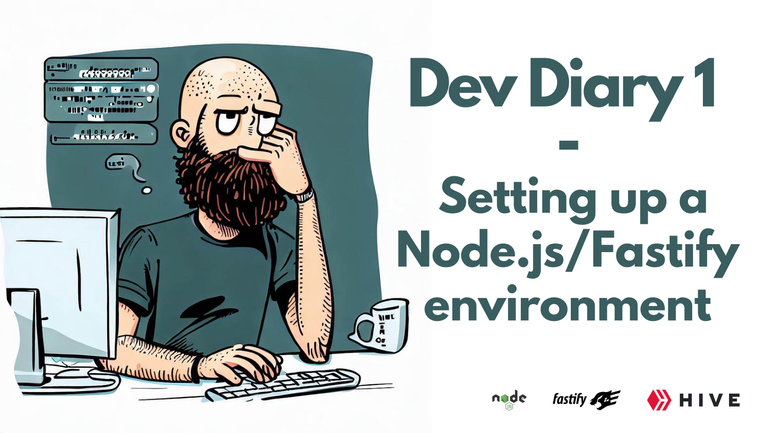
Image generated by Bing Image Creator
Reading my previous post (mentioned a the top) is a good introduction to this dev diary.
Choosing the right node version
I want my application to be easily deployable as a GCP App Engine. This requirement will limit the versions of node.js I can use.
The GCP App Engine documentation GCP mentions every even version since v10. Version 20 is in preview at the moment, but I will probably take a long time to develop and it will probably be GA before I finish.
Node.js 20 it is then!
I use nvm (node version manager) to handle my node versions.
$ nvm install v20.1.0
$ nvm use 20.1.0
The repo
I will push on a git personal repository. Nothing fancy to explain here. I instantiated the repo on GitHub and chose a private repo with a "node" .gitignore.
Directories
Let's keep it KISS :
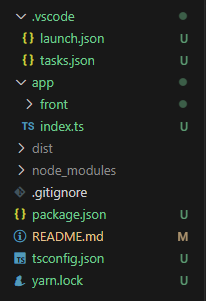
I need :
- an app directory with my code
- the server will be in this directory
- a front directory inside the app dir, for the frontend
- a dist directory which will hold the javascript generated by tsc (it's auto-generated)
- the .vscode directory for the VSCode configuration
- the classic files :
- package.json
- tsconfig.json (for tsc)
- yarn.lock (generated by yarn)
TSC Configuration
As I'll be coding in TypeScript, I need to translate TypeScript to JavaScript using TSC (The TypeScript Compiler). It will need some configuration. You can run
npx tsc --init
which will init a tsconfig.json file. It's useful as it will ask you some questions and the tsconfig.json file will have most of the possible variables commented on.
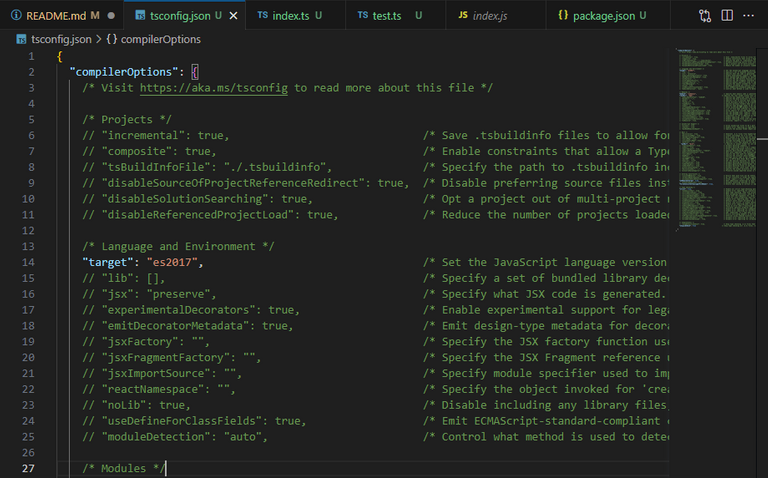
The generated tsconfig.json file
I had to change :
- "target": "es2017" => ES2017 is the minimal version to avoid the "FastifyDeprecation" message
- "rootDir": "app",
- "outDir": "dist",
I found the first point on the fastify website. The 2 others are your choice. They specify where your Typescript code is located and where the Javascript code should be generated.
Package.json
Nothing unusual in the package.json. I defined my basic scripts :
"scripts": {
"build": "tsc -p tsconfig.json",
"watch": "tsc -watch -p tsconfig.json",
"start": "node dist/index.js"
}
'Build' will run tsc and generate the Javascript files.
'Watch' does the same but 'listen' to your code change and update the files on the go
'Start' is the starting command for the server
VSCode configuration
This is based on an older project of mine, so I won't provide sources.
The config files go into the .vscode directory.
We first need a launch.json file
{
"version": "0.2.0",
"configurations": [
{
"type": "node",
"request": "launch",
"name": "Launch Program",
"skipFiles": [
"<node_internals>/**"
],
"cwd": "${workspaceRoot}/dist",
"program": "index.js",
"preLaunchTask": "npm: watch"
}
]
}
There's only one config at the time. It will execute the index.js file when you type 'F5' in an interactive debug session. I set the current working dir at the dist directory.
The preLaunchTask is important here. As you can see, I run the program in the dist directory. It's the directory containing the JavaScript files. It means that I need to have the most recent "compiled" files. So, I set a requirement to have the 'npm: watch' task running before starting the program.
For this preLaunchTask to be correctly handled by VSCode, we need to define a "task" in the tasks.json file:
{
"version": "2.0.0",
"tasks": [
{
"type": "npm",
"script": "watch",
"group": {
"kind": "build",
"isDefault": true
},
"problemMatcher": "$tsc-watch",
"label": "npm: watch",
"isBackground": true,
"detail": "tsc -watch"
}
]
}
I can't give more explanation here as it's a code I found a long time ago on the web. Anyway, it works as expected.
When you press 'F5', a dedicated terminal will open and tsc will run in watch mode, updating the Javascript file on the go, while you are writing your Typescript code, and the code will run in debug mode.
The code
I always start simple. The tutorial is more than enough to test if it works.
import fastify from 'fastify'
const server = fastify();
server.get('/ping', async (request, reply) => {
return 'pong !\n'
});
server.listen({ port: 8080, host: '0.0.0.0' }, (err, address) => {
if (err) {
console.error(err);
process.exit(1);
}
console.log(`Server listening at ${address}`);
});
The code is straightforward:
- import fastify
- instantiate a fastify server
- if the path is "myserver/ping" write 'pong'
- listen to port 8080, on all interfaces (host: '0.0.0.0')
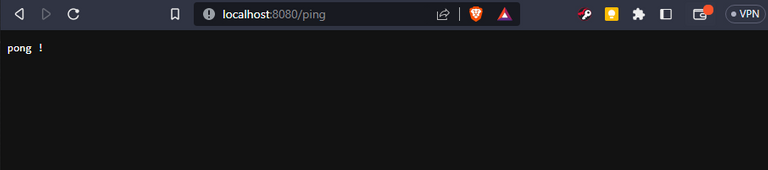
Alright!
Next steps :
The typescript tutorial uses the "await/async" version. I would prefer using the 'Promise' version. I still struggle to perfectly understand and use Promises in Node.js, so it will be a very good exercise.
I must have a look at the server creation parameters, to see if something is interesting or useful there. I already noticed a "logger: true" parameter that needs some interest.
Finally, I have to read about the "Plugins" and "Generics" that are mentioned in the documentation. I'll cover them in the next post.
Conclusions
- The setup was easy and I did not meet any problems.
- Writing the post still feels longer than the coding part.
- I did not code much in the last 2 weeks as I'm now on holiday and my time is better spent walking in the forest with my wife and my dog!
Thumbnail image generated by Bing Image Creator, modified with Canva.
Prompt : "a bald developer with a beard wondering how to make its code work, Belgian comic strip style"